Java, C#, Symfony, Laravel, Android, Xcode, Arduino Please Donate: https://streamlabs.com/georgesqhk
Sunday, March 16, 2014
Java Programming: CRUD Operations in laravel 4 with mysql
Java Programming: CRUD Operations in laravel 4 with mysql: CRUD Operations in Laravel 4 with MYSQL 1. INTRODUCTION Sometimes we need to create a CRUD operation for our projects, Create, Read, Upd...
CRUD Operations in laravel 4 with mysql
CRUD Operations in Laravel 4 with MYSQL
1. INTRODUCTION
Sometimes we need to create a CRUD operation for our projects, Create, Read, Update and Delete tables in database using Laravel. I admit it, Laravel is a complex framework, but once you understand how to manage it, it become easy and powerful framework for PHP development.At the end of this tutorial, you should be able to create a basic application where you can add, edit, delete and list Books.
2. CREATE A LARAVEL PROJECT
Create a project called bookshop3. CREATE TABLE
Before getting started, be sure to configure a database connection in app/config/database.php Just modify mysql connection.'mysql' => array( 'driver' => 'mysql', 'host' => 'localhost', 'database' => 'library', 'username' => 'root', 'password' => 'password', 'charset' => 'utf8', 'collation' => 'utf8_unicode_ci', 'prefix' => '', ),Using artisan, lets create a table and after that we will migrate to mysql database. For this, just open the console inside the project and type this line of command:
php artisan migrate:make create_books_table --create=books
This will create a php file in app/database/migrations.
The file have this name:
"####_##_##_######_create_books_table.php"
Open the file and add fields (id, isbn, title, author, publisher, language)
<?php use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateBooksTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { //create table in the database Schema::create('books',function(Blueprint $table) { $table->increments("id"); $table->string('isbn'); $table->string('title'); $table->string('author'); $table->string('publisher'); $table->integer('language'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { //drop table if exist in the database Schema::drop('books'); } }Now we need to migrate all this information into our database in the command line type:
php artisan migrate
Once you have executed the above command, this will create a table called books in our MYSQL database (no need to create manually books table, this is one of the artisan's advantages).
4. Create eloquent books model
The Eloquent ORM included with Laravel provides a beautiful, simple ActiveRecord implementation for working with your database. Each database table has a corresponding "Model" which is used to interact with that table. Now that we have our database, let’s create a simple book Eloquent model so that we can access the books in our database easily.Let's go to app/models folder and create a Books.php model and add the follow code(later we will add some more code to this model)
<?php class Book extends Eloquent { }
5. Create Book Controller
Let's create BookController, we can use artisan command for create it, in the command line type:php artisan controller:make BookController
<?php class BookController extends \BaseController { /** * Display a listing of the resource. * * @return Response */ public function index() { // } /** * Show the form for creating a new resource. * * @return Response */ public function create() { // } /** * Store a newly created resource in storage. * * @return Response */ public function store() { // } /** * Display the specified resource. * * @param int $id * @return Response */ public function show($id) { // } /** * Show the form for editing the specified resource. * * @param int $id * @return Response */ public function edit($id) { // } /** * Update the specified resource in storage. * * @param int $id * @return Response */ public function update($id) { // } /** * Remove the specified resource from storage. * * @param int $id * @return Response */ public function destroy($id) { // } }As we can see it create our BookController controller with all the methods we need, we need to implement it part by part.
6. Create routes for our Book CRUD
What Laravel provides us is that we can simply tap into either a GET or POST request via routes and send it to the appropriate method. Here is an example for that:Route::get('/register', 'BookController@showBookRegistration');
Route::post('/register', 'BookController@saveBook');
See the difference here is we are registering the same URL, /register, but we are defining its GET method so Laravel can call BookController class' showBookRegistration method. If it's the POST method, Laravel should call the saveBook method of the BookController class.
So let's create our route at /app/routes.php. Add the following line to the routes.php file: Route::resource('books','BookController');
<?php Route::get('/', function() { return View::make('hello'); }); Route::resource('books','BookController');You can also use the following command to view the list of all the routes in your project from the root of your project, launching command line:
php artisan routes
As you can see, resource Controller really makes your work easy. You don't have to create lots of routes.
7. Create view to show all books
Before create a view for show books, we need to know about Blade. Blade is a templating engine provided by Laravel. Blade has a very simple syntax, and you can determine most of the Blade expressions within your view files as they begin with "@".Blade syntax: {{ $var }}
PHP syntax:<?php echo $var; ?>
As we can see blade syntax is compact and easy to write.
In this section we will create blade templates and how to use it; and need to know that all Blade templates should use the .blade.php extension.
Now let's try to fetch books from our database via the Eloquent object. For this let's modify the index method in our app/controllers/BooksController.php.
public function index() { //get all Books $booksList=Book::all(); return View::make('books.index',compact('booksList')); }Where books are the folder in app/views/ and index will be the main page to show all books
Now let's create our template, create a folder called layouts in app/views and inside the new folder create a new file called book.blade.php and add the following code.
<!doctype html> <html> <head> <title>George's BookShop::Peru</title> <meta charset="utf-8"> <link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css"> <style> table form { margin-bottom: 0; } form ul { margin-left: 0; list-style: none; } .error { color: red; font-style: italic; } body { padding-top: 20px; } </style> </head> <body> <div class="container"> @if (Session::has('message')) <div class="flash alert"> <p>{{ Session::get('message') }}</p> </div> @endif @yield('main') </div> </body> </html>Now let's create the view for showing books, for this create a folder called books inside app/views and inside the new folder create a file called index.blade.php, and add the following code:
@extends('layouts/book') @section('main') <h1>Welcome to my bookshop</h1> <p>{{ link_to_route('books.create', 'Create new book') }}</p> @if ($booksList->count()) <table class="table table-striped table-bordered"> <thead> <tr> <th>Id</th> <th>ISBN</th> <th>Title</th> <th>Author</th> <th>Publisher</th> <th>Language</th> </tr> </thead> <tbody> @foreach ($booksList as $book) <tr> <td>{{ $book->id }}</td> <td>{{ $book->isbn }}</td> <td>{{ $book->title }}</td> <td>{{ $book->author }}</td> <td>{{ $book->publisher }}</td> <td>@if ($book->language==1){{'English'}} @else{{'Spanish'}} @endif</td> <td>{{ link_to_route('books.show', 'Read', array($book->id), array('class' => 'btn btn-primary')) }}</td> <td>{{ link_to_route('books.edit', 'Update', array($book->id), array('class' => 'btn btn-warning')) }}</td> <td> {{ Form::open(array('method'=> 'DELETE', 'route' => array('books.destroy', $book->id))) }} {{ Form::submit('Delete', array('class' => 'btn btn-danger')) }} {{ Form::close() }} </td> </tr> @endforeach </tbody> </table> @else There are no books @endif @stopIf we have followed the complete tutorial until this part so means our index page should work, but first, we need to add a new row in books table, this just for testing, later we will implement "add new book". After this let's start our server with the following command inside our bookshop project:
php artisan serve
As we can see, our server start to work
Let's open a brower and go to our books view and bravo!!!! It works :P
8. Create new Book
Now as we have listed our books, let's write the code for creating new book. To create a new book, we will need to create the Controller method and bind the view for displaying the new book form, so let's modify our BookController in /app/controllers/BookController.php and add just one line of code in create method:public function create() { //add new book form return View::make('books.create'); }We know that this is calling create view which still not yet implemented, so let's create the a new file called create.blade.php inside /app/views/books and lets add the following code:
@extends('layouts.book') @section('main') <h1>Create Book</h1> {{ Form::open(array('route' => 'books.store')) }} <ul> <li> {{ Form::label('ISBN', 'ISBN:') }} {{ Form::text('isbn') }} </li> <li> {{ Form::label('Title', 'Title:') }} {{ Form::text('title') }} </li> <li> {{ Form::label('Author', 'Author:') }} {{ Form::text('author') }} </li> <li> {{ Form::label('Publisher', 'Publisher:') }} {{ Form::text('publisher') }} </li> <li> {{ Form::label('Language', 'Language') }} {{ Form::select('language', array('0' => 'Select a Level', '1' => 'English', '2' => 'Spanish'), Input::old('language'), array('class' => 'form-control')) }} </li> <li> {{ Form::submit('Save', array('class' => 'btn btn-primary')) }} </li> </ul> {{ Form::close() }} @if ($errors->any()) <ul> {{ implode('', $errors->all('<li class="error">:message</li>')) }} </ul> @endif @stopLet's modify our Book Model /app/Models/Book.php, because our model needs to have the $fillable variable set, this variable is used to prevent mass assignment. See the documentation on mass-assignment for details.
<?php class Book extends Eloquent{ protected $fillable = array('isbn', 'title','author','publisher','language'); }Now let's create our store method to save our book form data into our books table, open /app/controllers/BookController.php and add the following code:
public function store() { //create a rule validation $rules=array( 'isbn'=>'required', 'title'=>'required', 'author'=>'required', 'publisher'=>'required', 'language'=>'required|numeric' ); //get all book information $bookInfo = Input::all(); //validate book information with the rules $validation=Validator::make($bookInfo,$rules); if($validation->passes()) { //save new book information in the database //and redirect to index page Book::create($bookInfo); return Redirect::route('books.index') ->withInput() ->withErrors($validation) ->with('message', 'Successfully created book.'); } //show error message return Redirect::route('books.create') ->withInput() ->withErrors($validation) ->with('message', 'Some fields are incomplete.'); }If we make click in Create New Book link we will redirect to create page depicted in the next figure:
9. Update Book information
Now as we learned how easy it is to add and list books. Let's jump into updating a book.In our list books view we have the Update link with the following code:{{ link_to_route('books.edit', 'Update', array($book->idbook), array('class' => 'btn btn-warning')) }}
Here, the link_to_route function will generate a link /books/<id>/edit, which will call the resourceful Controller book, and Controller will bind it with the edit method, so let's modify edit and update methods in our BookController /app/controllers/BookController:
public function edit($id) { //get the current book by id $book = Book::find($id); if (is_null($book)) { return Redirect::route('books.index'); } //redirect to update form return View::make('books.edit', compact('book')); } public function update($id) { //create a rule validation $rules=array( 'isbn'=>'required', 'title'=>'required', 'author'=>'required', 'publisher'=>'required', 'language'=>'required|numeric' ); $bookInfo = Input::all(); $validation = Validator::make($bookInfo, $rules); if ($validation->passes()) { $book = Book::find($id); $book->update($bookInfo); return Redirect::route('books.index') ->withInput() ->withErrors($validation) ->with('message', 'Successfully updated Book.'); } return Redirect::route('books.edit', $id) ->withInput() ->withErrors($validation) ->with('message', 'There were validation errors.'); }Now let's create our book edit view at /app/views/books/edit.blade.php, as follows:
@extends('layouts.book') @section('main') <h1>Edit Book</h1> {{ Form::model($book, array('method' => 'PATCH', 'route' =>array('books.update', $book->id))) }} <ul> <li> {{ Form::label('ISBN', 'ISBN:') }} {{ Form::text('isbn') }} </li> <li> {{ Form::label('Title', 'Title:') }} {{ Form::text('title') }} </li> <li> {{ Form::label('Author', 'Author:') }} {{ Form::text('author') }} </li> <li> {{ Form::label('Publisher', 'Publisher:') }} {{ Form::text('publisher') }} </li> <li> {{ Form::label('Language', 'Language') }} {{ Form::select('language', array('0' => 'Select a Level', '1' => 'English', '2' => 'Spanish'), null, array('class' => 'form-control')) }} </li> <li> {{ Form::submit('Update', array('class' => 'btn btn-warning'))}} {{ link_to_route('books.show', 'Cancel', $book-> id,array('class' => 'btn btn-danger')) }} </li> </ul> {{ Form::close() }} @if ($errors->any()) <ul> {{implode('',$errors->all('<li class="error">:message</li>'))}} </ul> @endif @stopAfter these modification our update function works, let's tried to update book information and we will get the next figure:
10. Delete Book
To delete a book we can use the destroy method. The same like update method If you go to our book lists view, you can find the following delete link's generation code:{{ Form::open(array('method' => 'DELETE', 'route' => array('books.destroy', $book->id))) }} {{ Form::submit('Delete', array('class' => 'btn btn-danger')) }} {{ Form::close() }}Let's go to BookController at /app/controllers/BookController.php this request will hit the destroy() method as follows:
public function destroy($id) { //delete book Book::find($id)->delete(); return Redirect::route('books.index') ->withInput() ->with('message', 'Successfully deleted Book.'); }Once we delete one book it will redirect to our index page and show message "Successfully deleted book" similar case in update function.
11. Pagination
We can add pagination to our CRUD book page like depicted in next picture:Tuesday, March 11, 2014
Java Programming: Instalacion y Configuracion de Laravel 4 en window...
Java Programming: Instalacion y Configuracion de Laravel 4 en window...: INSTALACION Y CONFIGURACION DE LARAVEL 4 WINDOWS 7 1. Introduccion Todos, al comenzar un proyecto web personal o profesional; siempre no...
Instalacion y Configuracion de Laravel 4 en windows 7
INSTALACION Y CONFIGURACION DE LARAVEL 4 WINDOWS 7
1. Introduccion
Todos, al comenzar un proyecto web personal o profesional; siempre nos preguntamos cuál es la forma más rápida y eficiente de lograrlo, que framework debemos utilizar. De estas preguntas dependen, las ganancias del proyecto y el futuro mantenimiento del mismo. Y casi siempre a la hora de probar alguno de estos frameworks(Symfony, Ruby on Rails, Sinatra, Laravel, etc) no vemos con la frustración de ver que no funciona siquiera un ejemplo básico a la hora de instalar, esto debido a que debe hacerse algunas configuraciones manuales en los archivos de PHP y del servidor WAMPSERVER. En este tutorial se pretende mostrar la forma correcta de instalar el framework laravel en un servidor WAMPSERVER y Windows 7 como sistema operativo.2. Que es Laravel 4
Laravel es un framework de código libre para desarrollar aplicaciones web con PHP el cual últimamente viene creciendo en popularidad por la comunidad de desarrolladores en PHP. Su filosofía es desarrollar código PHP de forma elegante y simple, evitando el "código espagueti". Fue creado en 2011 y tiene una gran influencia de frameworks como Ruby on Rails, Sinatra, Symfony y ASP.NET MVC.3. Requisitos para el funcionamiento de laravel
- WAMP Server
Es un entorno para el desarrollo web utilizado en el sistema operativo windows; ya que funciona al igual como si cuando trabajamos en un servidor web, ya que podemos ejecutar estas aplicaciones web de manera local y ver como seria el funcionamiento antes de ser subidas a un hosting o servidor web. Además de ello podemos gestionar datos con la ayuda del motor de base de datos (MySQL) y su administrador (PHPMyAdmin). - Composer
Composer es un manejador de dependencias para PHP( por linea de comandos), este proyecto inspirado en npm y Bundler y sirve para administrar librerías de terceros o propias en nuestros proyectos de PHP a la hora de trabajar con laravel.
4. Instalar WAMP server
Descargar Wamp Server de la página oficialhttp://www.wampserver.com/en/
para la demostración de este tutorial se utilizo la versión 2.4, installar en el directorio por defecto "c:/wamp" Luego de haber instalado wampserver, abrir un navegador web comprobar que nuestro servidor wampserver se encuentra en funcionamiento, tal como se muestra en la figura siguiente:
5. Configuracion de Wamp Server
Antes de pasar a la instalacion de laravel, debemos configurar algunos archivos de PHP y de Apache en el servidor Wamp( Ojo si no se configura estos archivos no funcionara los siguientes pasos, ya que composer emitira un error al momento de instalacion tal como se indica a continuacion)Error when installing composer
"Some settings on your machine make Composer unable to work properly. Make sure that you fix the issues listed below and run this script again: The openssl extension is missing, which means that secure HTTPS transfers are impossible. If possible you should enable it or recompile php with --with-openssl".
- Dirigirse a C:\wamp\bin\php\php5.4.16 y editar el archivo php.ini, descomentar extension=php_openssl.dll
- Digirirse a C:\wamp\bin\apache\Apache2.4.4\bin y editar el archivo php.ini y descomentar extension=php_openssl.dll
6. Instalar Composer
Descargar Composer de su pagina oficial https://getcomposer.org/ y luego de descargar doble click para empezar su instalacion, luego hacer Click en Next.Si deseas administrar tus proyectos mediante el windows explorer puedes selecionar la opcion "Install Shell Menus" aunque lo recomendable es la administracion por la linea de comandos.
A continuacion nos pedira que selecionemos el archivo de ejecucion de PHP, para esto dirigirse a C:\wamp\bin\php\php5.4.16 y seleccionar php.exe, luego click en Next.
Luego nos pedira el navegador que utilizaremos por defecto, seleccionar el navegador de tu preferencia y luego click en Next y completar la instalacion.
Para comprobar que realmente tenemos instalado correctamente composer, abrir un terminal de windows y escribir composer y presionar enter, nos debera mostrar el logo de composer tal como se muestra en la figura siguiente:
7. Crear un proyecto laravel con composer
Existen dos formas de crear un proyecto con laravel, uno es descargando el archivo master de internet y la otra es mediante composer tal como lo haremos a continuacion.Para crear un proyecto web con laravel 4 abrimos un terminal de windows y dirigirse a C:/wamp/www: Crear un nuevo directorio el cual sera un proyecto de laravel para esto simplemente digitar mkdir laraveltest y presionar enter; luego acceder a dicho directorio y ahora crearemos el proyecto laravel escribiendo lo siguiente:
composer create-project laravel/laravel --prefer-dist
Luego de esto empezara a descargar las librerias necesarias para nuestro proyecto laravel. En la siguiente figura se muestra todo lo explicado en esta parte.
Si no hubo problemas de conexion con internet, nos mostrara la ventana siguiente donde nos indica que nuestro proyecto se creo correctamente.
8. Ejecucion del proyecto creado
Antes de ejecutar nuestro proyecto laraval creado anteriormente agreguemos algo al archivo hello.php el cual se encuentra ubicado en c:\wamp\www\laraveltest\laravel\app\views Abrir con cualquier editor de texto y agregar una etiqueta h1 con tu nombreAbrir un navegador web y escribir lo siguiente: http://localhost/laraveltest/laravel/public/
Wednesday, March 5, 2014
Windows Communication Foundation vs Web Services asmx
WCF vs Web Services ASMX
What is WCF?
Windows Communication Foundation (WCF) is a unified framework for creating secure, reliable, transacted, and interoperable distributed applications. In earlier versions of Visual Studio, there were several technologies that could be used for communicating between applications(example web services asmx).What is Web Services ASMX
ASMX web services has been the favorite choice for many developers for building soap web services in .NET during a long time because of its simplicity. With ASMX web services, you get a web service up and running in few simple steps, as it does not require any configuration. The only thing you need to do is to build the service implementation and the message contracts.WCF vs Web Service ASMX
WCF | Web Services ASMX |
|
|
How to create an Web Service ASMX
File -> New ->Web Site, choose any name you like for my example WebASMX, after create project, let's add a new web services asmx, right click on the project and then Add - New Item and choose Web Service(ASMX) and change the name to WebService.asmx.
As we can see the new web service is empty as we can see in the below source code.
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Services; ///Let's continue adding a new WebMethod to our new web service asmx, for example a simple method which can add 2 numbers, pay attention that all web services need to have WebMethod between brackets./// Summary description for WebService /// [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] // To allow this Web Service to be called from script, using ASP.NET // AJAX, uncomment the following line. // [System.Web.Script.Services.ScriptService] public class WebService : System.Web.Services.WebService { public WebService () { //Uncomment the following line if using designed components //InitializeComponent(); } [WebMethod] public string HelloWorld() { return "Hello World"; } }
[WebMethod] public int Add(int a, int b) { return a + b; }
Testing the web Services
Press F5 to run the web services asmx; a web form will be displayed and it will load the web services available in our web service; in our example it show Add and HelloWorld web services; just make click on the link and Let's enter 2 numbers and click in Invoke button and it will be displayed another window where it shown the result..
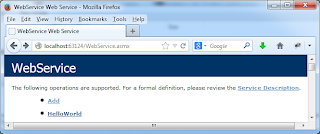
Accessing the Web Service asmx
Let's create a windows project to test our simple web service asmx File -> Add New project and I choose the name wsAddNumbers as its name and Add some basic controls to the project to add 2 numbers as shown next figure.
Add Web Service ASMX reference to our client
Let's locate in our project; right click on reference -> Add Service Reference and it will be displayed a window where we need to choose our web service, once you choose the web service from the left side, it will display all the methods of the web services in the right side as shown in next figure:
To build a client application
In our windows Form double-click in Add button, and add the following code in the click event handler, first make sure you added the web reference to your project.private void btnAdd_Click(object sender, EventArgs e) { ServiceReference1.WebServiceSoap ws= new ServiceReference1.WebServiceSoapClient(); int a = Int16.Parse(txtNumber1.Text); int b = Int16.Parse(txtNumber2.Text); int r = ws.Add(a, b); txtResult.Text = r.ToString(); }
Running our Web Service ASMX client
Press F5 to run and enter 2 numbers and click in Add button and see the resultsHow to Create a WCF
In this section we will create the new WCF service which will replace soon the Web service asmx. On the file -> New Project Click in WCF, followed by WCF Service Library. Chooese a name WcfServiceLibraryAdd Click OK to open the project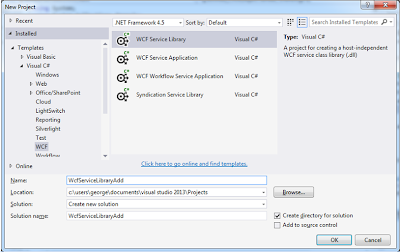
As we can see IService1 class is more structured compared with web service asmx, also can see clearly the interface service where we will add a new OperationContract
private void btnAdd_Click(object sender, EventArgs e) { using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace WcfServiceLibraryAdd { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "IService1" in both code and config file together. [ServiceContract] public interface IService1 { [OperationContract] string GetData(int value); [OperationContract] CompositeType GetDataUsingDataContract(CompositeType composite); // TODO: Add your service operations here } // Use a data contract as illustrated in the sample below to add composite types to service operations. // You can add XSD files into the project. After building the project, you can directly use the data types defined there, with the namespace "WcfServiceLibraryAdd.ContractType". [DataContract] public class CompositeType { bool boolValue = true; string stringValue = "Hello "; [DataMember] public bool BoolValue { get { return boolValue; } set { boolValue = value; } } [DataMember] public string StringValue { get { return stringValue; } set { stringValue = value; } } } } }Let's add a new OperationContract to Add two numbers Go to IService1.cs and add a new operation to the interface as shown in the code.
[ServiceContract] public interface IService1 { [OperationContract] string GetData(int value); [OperationContract] int Add(int a, int b); [OperationContract] CompositeType GetDataUsingDataContract(CompositeType composite); // TODO: Add your service operations here }Go to the Service1.cs class and implement the new operation as shown in next code.
using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace WcfServiceLibraryAdd { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the class name "Service1" in both code and config file together. public class Service1 : IService1 { public string GetData(int value) { return string.Format("You entered: {0}", value); } public int Add(int a, int b) { return a + b; } ...........
Testing WCF Service
Press F5 and choose the method we implemented in this case "Add" function; enter 2 numbers and click Invoke, as you can see all the procedure is show in the same window, don't worry if you see some error cross in some method, this is normal.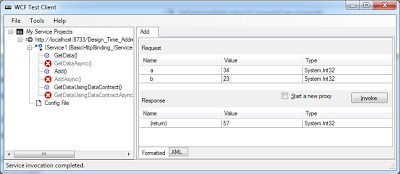
Accessing the WCF Service
Lets create a simple windows form like we did for testing web services asmx; you can reuse the same project in order to save time, just make sure to delete the import libraries.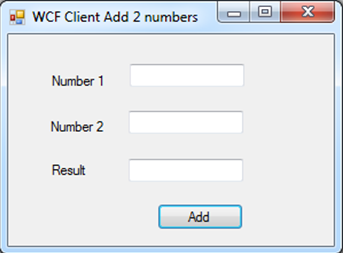
Add Service Reference
Right-click in the project and click Add Service Reference. Add Service Reference dialog box will appear ( if doesn’t appear once you click in Discover, copy and paste the address directly and click Go) this because our WCF is not in real web server, just running in virtual server.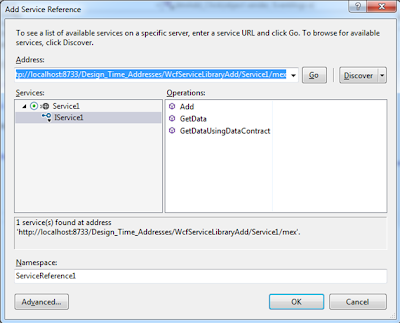
Build the WCF Client Application
Double click Add button and add the following code; just make sure you add the reference to WCF service.private void btnAdd_Click(object sender, EventArgs e) { ServiceReference1.Service1Client client = new ServiceReference1.Service1Client(); int a=Int16.Parse(txtNumber1.Text); int b=Int16.Parse(txtNumber2.Text); int result = client.Add(a, b); txtResult.Text = result.ToString(); }
Running our Web Service WCF client
Press F5, enter 2 numbers and press Add button.<?php $example = range(0, 9); foreach ($example as $value) { echo $value; }
Subscribe to:
Posts (Atom)