WCF vs Web Services ASMX
What is WCF?
Windows Communication Foundation (WCF) is a unified framework for creating secure, reliable, transacted, and interoperable distributed applications. In earlier versions of Visual Studio, there were several technologies that could be used for communicating between applications(example web services asmx).What is Web Services ASMX
ASMX web services has been the favorite choice for many developers for building soap web services in .NET during a long time because of its simplicity. With ASMX web services, you get a web service up and running in few simple steps, as it does not require any configuration. The only thing you need to do is to build the service implementation and the message contracts.WCF vs Web Service ASMX
WCF | Web Services ASMX |
|
|
How to create an Web Service ASMX
File -> New ->Web Site, choose any name you like for my example WebASMX, after create project, let's add a new web services asmx, right click on the project and then Add - New Item and choose Web Service(ASMX) and change the name to WebService.asmx.
As we can see the new web service is empty as we can see in the below source code.
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Services; ///Let's continue adding a new WebMethod to our new web service asmx, for example a simple method which can add 2 numbers, pay attention that all web services need to have WebMethod between brackets./// Summary description for WebService /// [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] // To allow this Web Service to be called from script, using ASP.NET // AJAX, uncomment the following line. // [System.Web.Script.Services.ScriptService] public class WebService : System.Web.Services.WebService { public WebService () { //Uncomment the following line if using designed components //InitializeComponent(); } [WebMethod] public string HelloWorld() { return "Hello World"; } }
[WebMethod] public int Add(int a, int b) { return a + b; }
Testing the web Services
Press F5 to run the web services asmx; a web form will be displayed and it will load the web services available in our web service; in our example it show Add and HelloWorld web services; just make click on the link and Let's enter 2 numbers and click in Invoke button and it will be displayed another window where it shown the result..
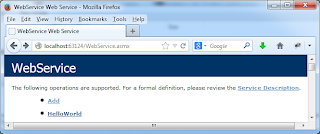
Accessing the Web Service asmx
Let's create a windows project to test our simple web service asmx File -> Add New project and I choose the name wsAddNumbers as its name and Add some basic controls to the project to add 2 numbers as shown next figure.
Add Web Service ASMX reference to our client
Let's locate in our project; right click on reference -> Add Service Reference and it will be displayed a window where we need to choose our web service, once you choose the web service from the left side, it will display all the methods of the web services in the right side as shown in next figure:
To build a client application
In our windows Form double-click in Add button, and add the following code in the click event handler, first make sure you added the web reference to your project.private void btnAdd_Click(object sender, EventArgs e) { ServiceReference1.WebServiceSoap ws= new ServiceReference1.WebServiceSoapClient(); int a = Int16.Parse(txtNumber1.Text); int b = Int16.Parse(txtNumber2.Text); int r = ws.Add(a, b); txtResult.Text = r.ToString(); }
Running our Web Service ASMX client
Press F5 to run and enter 2 numbers and click in Add button and see the resultsHow to Create a WCF
In this section we will create the new WCF service which will replace soon the Web service asmx. On the file -> New Project Click in WCF, followed by WCF Service Library. Chooese a name WcfServiceLibraryAdd Click OK to open the project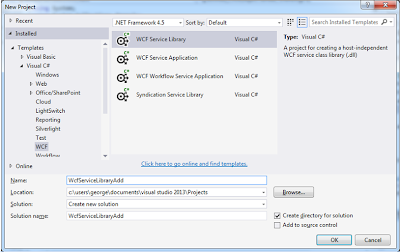
As we can see IService1 class is more structured compared with web service asmx, also can see clearly the interface service where we will add a new OperationContract
private void btnAdd_Click(object sender, EventArgs e) { using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace WcfServiceLibraryAdd { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "IService1" in both code and config file together. [ServiceContract] public interface IService1 { [OperationContract] string GetData(int value); [OperationContract] CompositeType GetDataUsingDataContract(CompositeType composite); // TODO: Add your service operations here } // Use a data contract as illustrated in the sample below to add composite types to service operations. // You can add XSD files into the project. After building the project, you can directly use the data types defined there, with the namespace "WcfServiceLibraryAdd.ContractType". [DataContract] public class CompositeType { bool boolValue = true; string stringValue = "Hello "; [DataMember] public bool BoolValue { get { return boolValue; } set { boolValue = value; } } [DataMember] public string StringValue { get { return stringValue; } set { stringValue = value; } } } } }Let's add a new OperationContract to Add two numbers Go to IService1.cs and add a new operation to the interface as shown in the code.
[ServiceContract] public interface IService1 { [OperationContract] string GetData(int value); [OperationContract] int Add(int a, int b); [OperationContract] CompositeType GetDataUsingDataContract(CompositeType composite); // TODO: Add your service operations here }Go to the Service1.cs class and implement the new operation as shown in next code.
using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace WcfServiceLibraryAdd { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the class name "Service1" in both code and config file together. public class Service1 : IService1 { public string GetData(int value) { return string.Format("You entered: {0}", value); } public int Add(int a, int b) { return a + b; } ...........
Testing WCF Service
Press F5 and choose the method we implemented in this case "Add" function; enter 2 numbers and click Invoke, as you can see all the procedure is show in the same window, don't worry if you see some error cross in some method, this is normal.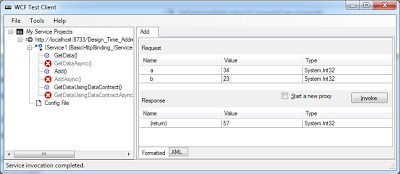
Accessing the WCF Service
Lets create a simple windows form like we did for testing web services asmx; you can reuse the same project in order to save time, just make sure to delete the import libraries.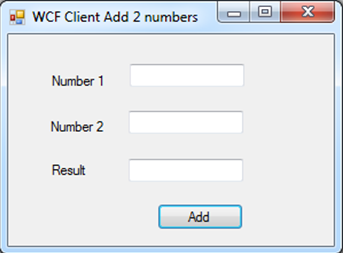
Add Service Reference
Right-click in the project and click Add Service Reference. Add Service Reference dialog box will appear ( if doesn’t appear once you click in Discover, copy and paste the address directly and click Go) this because our WCF is not in real web server, just running in virtual server.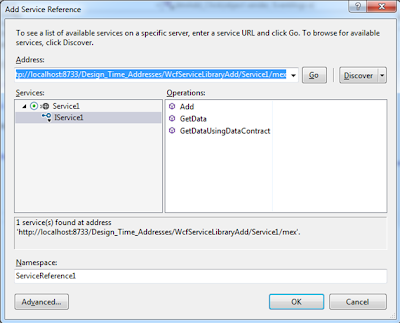
Build the WCF Client Application
Double click Add button and add the following code; just make sure you add the reference to WCF service.private void btnAdd_Click(object sender, EventArgs e) { ServiceReference1.Service1Client client = new ServiceReference1.Service1Client(); int a=Int16.Parse(txtNumber1.Text); int b=Int16.Parse(txtNumber2.Text); int result = client.Add(a, b); txtResult.Text = result.ToString(); }
Running our Web Service WCF client
Press F5, enter 2 numbers and press Add button.<?php $example = range(0, 9); foreach ($example as $value) { echo $value; }
this is a test
ReplyDeleteBut this is very interesting. well congratulations
ReplyDeleteFor more information on Web Development company, check out the info available online; these will help you learn to find the Website Design company.
ReplyDeleteThis is very interesting... Anar .NET Solutions
ReplyDelete
ReplyDeleteBlog really helpful for us
Thanks
Online affiliates